Mastering C++: Essential Skills Every Developer Should Know
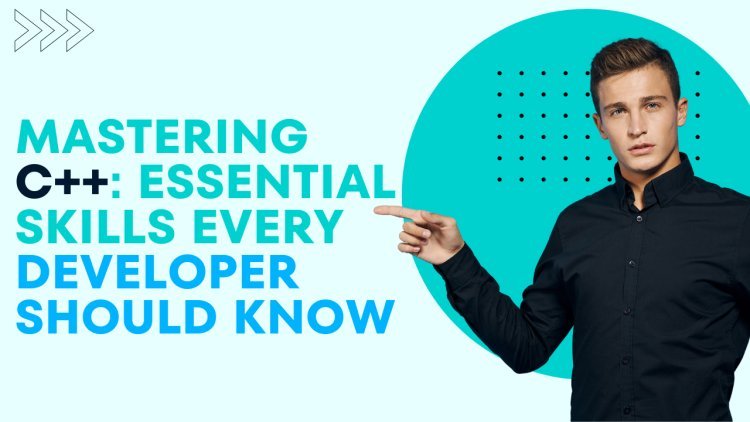
C++ is a versatile programming language that has stood the test of time, blending both high and low-level programming features. Whether you're a budding programmer or a seasoned developer, mastering C++ can open up numerous doors in software development, game programming, and systems-level coding. If you’re also familiar with the C++ String functionalities, you’ll find that C++ offers a robust set of tools to manipulate strings just as effectively as its counterparts in other languages, such as the Java String Method.
In this article, we’ll delve into the essential skills every C++ developer should know to elevate their programming game. From fundamental concepts to advanced techniques, we’ll cover a variety of topics that are critical for success in C++. Let’s jump right in!
Understanding the Basics of C++
Why C++?
C++ has a rich history dating back to the early 1980s and has evolved into one of the most widely used programming languages today. Its ability to support both object-oriented and procedural programming makes it an excellent choice for a variety of applications, including:
- Game development
- Operating systems
- Embedded systems
- High-performance applications
Key Features of C++
To effectively master C++, you should familiarize yourself with its core features:
- Object-Oriented Programming (OOP): Understanding classes and objects.
- Memory Management: Using pointers and dynamic memory allocation.
- Standard Template Library (STL): Utilizing templates and data structures.
Setting Up Your Development Environment
Before diving into C++, you need to set up your development environment. Popular IDEs like Visual Studio, Code::Blocks, and CLion provide powerful tools for writing and debugging your code. Installing a compiler like GCC or Clang is also essential for compiling your programs efficiently.
Essential Skills for Mastering C++
1. Proficiency in Syntax and Data Types
C++ syntax can be tricky for beginners. It’s crucial to become comfortable with the following data types:
- Primitive Types: int, char, float, double
- Compound Types: arrays, structs, unions
- User-defined Types: classes, enums
Understanding how these data types work will lay the foundation for writing effective code.
2. Mastering Control Structures
Control structures dictate the flow of your program. Familiarity with these structures will help you write more efficient and readable code.
Conditional Statements
- if-else
- switch-case
Looping Constructs
- for loops
- while loops
- do-while loops
Using these control structures effectively will enable you to make decisions and repeat actions within your programs.
3. Working with Functions
Functions are crucial in C++ for organizing code and promoting reusability. You should understand:
- Function Declaration: How to declare a function.
- Function Overloading: Using the same function name for different types or numbers of parameters.
By mastering functions, you’ll write cleaner and more modular code.
4. Object-Oriented Programming (OOP)
OOP is at the heart of C++. Understanding the four main principles—encapsulation, inheritance, polymorphism, and abstraction—is key to mastering this language.
Encapsulation
This principle restricts access to certain components of an object, allowing only necessary interactions. It promotes data hiding and keeps your code organized.
Inheritance
Inheritance allows one class to inherit the properties and methods of another, promoting code reuse. For instance, a Car class could inherit from a more general Vehicle class.
Polymorphism
Polymorphism enables a single interface to represent different types. You can achieve this through function overloading and operator overloading.
Abstraction
Abstraction focuses on hiding complex realities while exposing only the necessary parts. This simplifies your code and makes it easier to understand.
5. Mastering C++ Strings
Strings in C++ are a fundamental aspect of programming, often used to handle text and manipulate user input. The C++ String class simplifies the process of managing strings.
Creating and Manipulating Strings
- Initialization: Use std::string to create strings.
- Concatenation: Combine strings using the + operator.
- Length: Determine string length using .length() or .size().
6. Memory Management
Understanding memory management is crucial in C++. You'll often deal with dynamic memory allocation using new and delete. Grasping how memory works in C++ helps prevent leaks and crashes.
7. The Standard Template Library (STL)
The STL is a powerful feature of C++ that provides ready-to-use classes and functions. It includes:
- Containers: Such as vectors, lists, and maps.
- Algorithms: Sorting, searching, and manipulating data.
Becoming proficient in the STL will save you time and make your code more efficient.
8. Exception Handling
Proper exception handling is essential for building robust applications. Familiarize yourself with:
- try, catch, and throw: These keywords are fundamental to handling errors gracefully.
- Standard exceptions: Such as std::runtime_error and std::invalid_argument.
Mastering exception handling will ensure your program can deal with unexpected situations effectively.
9. Advanced Features: Templates and Lambda Functions
As you grow more comfortable with C++, you'll encounter advanced features that can enhance your programming:
Templates
Templates allow you to write generic and reusable code. They enable you to create functions and classes that can operate with any data type.
Lambda Functions
Introduced in C++11, lambda functions provide a way to define anonymous functions in a concise manner. They are especially useful for short snippets of code that are only needed temporarily.
Real-World Applications of C++
C++ is not just a theoretical language; it’s used in a variety of real-world applications. Understanding these applications can provide you with insight into its capabilities:
- Game Development: Many game engines, like Unreal Engine, are built using C++.
- System Software: Operating systems like Windows and Linux have portions written in C++.
- Web Browsers: Browsers such as Chrome and Firefox use C++ for performance-critical components.
Conclusion
Mastering C++ requires dedication, practice, and a willingness to dive deep into its concepts and features. By focusing on essential skills like syntax, OOP, memory management, and the Standard Template Library, you can set yourself up for success in your programming journey. Remember, understanding the C++ String functionalities and comparing them with something like the Java String Method can also enrich your programming perspective.
As you continue to hone your C++ skills, keep experimenting with new projects and challenges. The world of C++ is vast, and each new skill you acquire will only enhance your capabilities as a developer. Happy coding!
FAQ:
Q1: How long does it take to master C++?
Mastering C++ can vary widely depending on your prior programming experience. Generally, with consistent practice, you can become proficient in six months to a year.
Q2: Is C++ still relevant today?
Absolutely! C++ is widely used in performance-critical applications, game development, and systems programming, making it highly relevant in today's tech landscape.
Q3: What resources are best for learning C++?
There are numerous resources, including online courses, books, and documentation. Websites like Codecademy, Udemy, and the official C++ documentation can be excellent starting points.
Q4: How does C++ compare to other languages?
C++ offers more control over system resources than many high-level languages. It combines low-level and high-level programming features, making it more versatile.
What's Your Reaction?






